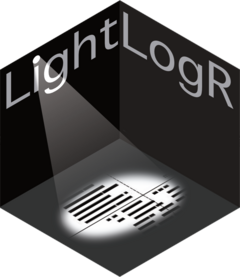
Find threshold for given duration
Source:R/metric_threshold_for_duration.R
threshold_for_duration.Rd
This function finds the threshold for which light levels are above/below for
a given duration. This function can be considered as the inverse of
duration_above_threshold
.
Usage
threshold_for_duration(
Light.vector,
Time.vector,
duration,
comparison = c("above", "below"),
epoch = "dominant.epoch",
na.rm = FALSE,
as.df = FALSE
)
Arguments
- Light.vector
Numeric vector containing the light data.
- Time.vector
Vector containing the time data. Can be POSIXct, hms, duration, or difftime.
- duration
The duration for which the threshold should be found. Can be either a duration or a string. If it is a string, it needs to be a valid duration string, e.g.,
"1 day"
or"10 sec"
.- comparison
String specifying whether light levels above or below the threshold should be considered. Can be either
"above"
(the default) or"below"
.- epoch
The epoch at which the data was sampled. Can be either a duration or a string. If it is a string, it needs to be either
"dominant.epoch"
(the default) for a guess based on the data, or a valid duration string, e.g.,"1 day"
or"10 sec"
.- na.rm
Logical. Should missing values (NA) be removed for the calculation? Defaults to
FALSE
.- as.df
Logical. Should a data frame with be returned? If
TRUE
, a data frame with a single column namedthreshold_{comparison}_for_{duration}
will be returned. Defaults toFALSE
.
See also
Other metrics:
bright_dark_period()
,
centroidLE()
,
disparity_index()
,
duration_above_threshold()
,
exponential_moving_average()
,
frequency_crossing_threshold()
,
interdaily_stability()
,
intradaily_variability()
,
midpointCE()
,
nvRC()
,
nvRD()
,
nvRD_cumulative_response()
,
period_above_threshold()
,
pulses_above_threshold()
,
timing_above_threshold()
Examples
N <- 60
# Dataset with 30 min < 250lx and 30min > 250lx
dataset1 <-
tibble::tibble(
Id = rep("A", N),
Datetime = lubridate::as_datetime(0) + lubridate::minutes(1:N),
MEDI = sample(c(sample(1:249, N / 2, replace = TRUE),
sample(250:1000, N / 2, replace = TRUE))),
)
dataset1 %>%
dplyr::reframe("Threshold above which for 30 mins" =
threshold_for_duration(MEDI, Datetime, duration = "30 mins"))
#> # A tibble: 1 × 1
#> `Threshold above which for 30 mins`
#> <int>
#> 1 291
dataset1 %>%
dplyr::reframe("Threshold below which for 30 mins" =
threshold_for_duration(MEDI, Datetime, duration = "30 mins",
comparison = "below"))
#> # A tibble: 1 × 1
#> `Threshold below which for 30 mins`
#> <int>
#> 1 248
dataset1 %>%
dplyr::reframe(threshold_for_duration(MEDI, Datetime, duration = "30 mins",
as.df = TRUE))
#> # A tibble: 1 × 1
#> threshold_above_for_30_minutes
#> <int>
#> 1 291