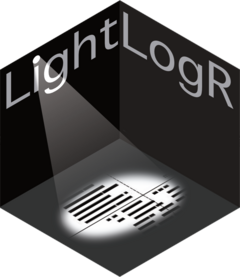
Duration above/below threshold or within threshold range
Source:R/metric_duration_above_threshold.R
duration_above_threshold.Rd
This function calculates the duration spent above/below a specified threshold light level or within a specified range of light levels.
Usage
duration_above_threshold(
Light.vector,
Time.vector,
comparison = c("above", "below"),
threshold,
epoch = "dominant.epoch",
na.rm = FALSE,
as.df = FALSE
)
Arguments
- Light.vector
Numeric vector containing the light data.
- Time.vector
Vector containing the time data. Can be POSIXct, hms, duration, or difftime.
- comparison
String specifying whether the time above or below threshold should be calculated. Can be either
"above"
(the default) or"below"
. If two values are provided forthreshold
, this argument will be ignored.- threshold
Single numeric value or two numeric values specifying the threshold light level(s) to compare with. If a vector with two values is provided, the time within the two thresholds will be calculated.
- epoch
The epoch at which the data was sampled. Can be either a duration or a string. If it is a string, it needs to be either
"dominant.epoch"
(the default) for a guess based on the data, or a valid duration string, e.g.,"1 day"
or"10 sec"
.- na.rm
Logical. Should missing values (NA) be removed for the calculation? Defaults to
FALSE
.- as.df
Logical. Should a data frame with be returned? If
TRUE
, a data frame with a single column namedduration_{comparison}_{threshold}
will be returned. Defaults toFALSE
.
Value
A duration object as single value, or single column data frame.
References
Hartmeyer, S.L., Andersen, M. (2023). Towards a framework for light-dosimetry studies: Quantification metrics. Lighting Research & Technology. doi:10.1177/14771535231170500
See also
Other metrics:
bright_dark_period()
,
centroidLE()
,
disparity_index()
,
dose()
,
exponential_moving_average()
,
frequency_crossing_threshold()
,
interdaily_stability()
,
intradaily_variability()
,
midpointCE()
,
nvRC()
,
nvRD()
,
nvRD_cumulative_response()
,
period_above_threshold()
,
pulses_above_threshold()
,
threshold_for_duration()
,
timing_above_threshold()
Examples
N <- 60
# Dataset with epoch = 1min
dataset1 <-
tibble::tibble(
Id = rep("A", N),
Datetime = lubridate::as_datetime(0) + lubridate::minutes(1:N),
MEDI = sample(c(sample(1:249, N / 2), sample(250:1000, N / 2))),
)
# Dataset with epoch = 30s
dataset2 <-
tibble::tibble(
Id = rep("B", N),
Datetime = lubridate::as_datetime(0) + lubridate::seconds(seq(30, N * 30, 30)),
MEDI = sample(c(sample(1:249, N / 2), sample(250:1000, N / 2))),
)
dataset.combined <- rbind(dataset1, dataset2)
dataset1 %>%
dplyr::reframe("TAT >250lx" = duration_above_threshold(MEDI, Datetime, threshold = 250))
#> # A tibble: 1 × 1
#> `TAT >250lx`
#> <Duration>
#> 1 1800s (~30 minutes)
dataset1 %>%
dplyr::reframe(duration_above_threshold(MEDI, Datetime, threshold = 250, as.df = TRUE))
#> # A tibble: 1 × 1
#> duration_above_250
#> <Duration>
#> 1 1800s (~30 minutes)
# Group by Id to account for different epochs
dataset.combined %>%
dplyr::group_by(Id) %>%
dplyr::reframe("TAT >250lx" = duration_above_threshold(MEDI, Datetime, threshold = 250))
#> # A tibble: 2 × 2
#> Id `TAT >250lx`
#> <chr> <Duration>
#> 1 A 1800s (~30 minutes)
#> 2 B 900s (~15 minutes)